Variables: A variable is simply a place to store data and it has a name and data type. In visual basic .net, a variable is declared using the Dim statement.
Syntax: Dim varName As varType
Here varName is the name of the variable.
varType is the datatype of the variable.
Data type: Data type define the type of data that a variable can store.
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------
VB alias .Net Type Size Range
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------
SByte System.SByte 8 bits -128 to 127
Byte System.Byte 8 bits 0 to 255
Short System. Int16 16 bits -32,768 to 32,767
UShort System.UInt16 16 bits 0 to 65,535
Integer System.Int32 32 bits -2147483648 to 2147483647
UInteger System.UInt32 32 bits 0 to 4294967295
Long System.Int64 64 bits -9223372036854775808 to 9223372036854775807
ULong System.UInt64 64 bits 0 to 18446744073709551615
Single System.Single 32 bits
Double System.Double 64 bits
Decimal System.Decimal 128 bits
Char System.Char 16 bits one unicode symbol in the range of 0 to 65535
Boolean System.Boolean 32 bits true or false
Object System.Object 32/64 bits platform dependent
Date System.DateTime 64 bits Jan 1,0001 12:00:00 am to Dec 31,9999 11:59:59 pm
String System.String 80+[16xLength]bits
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Variable Initialization: Variables are initialized with an equal sign followed by a constant expression. The general form of initialization is.
ex: Dim pi As Double
pi=3.14159
Accepting values from user: The Console class in the system namespace provides a function ReadLine for accepting input from the user and store it into a variable.
ex: Dim message As String
message = Console.ReadLine
Declaring Constants: Constants are declared using the Const statement. The const statement is used at module, class, structure, procedure or block level for use in place of literal values.
syntax: constantname [ As datatype ] = initializer
Print and Display constants:
vbNewLine : new line character.
vbNullChar : null character.
vbNullString : not the same as a zero length stirng, used for calling external procedures.
vbTab : tab character.
vbBack : backspace character.
vbCrLf : carriage return/linefeed character combination.
vbCr : carriage return character.
vbLf : Linefeed character.
Modifiers: The modifiers are keywords added with any programming element to give some especial emphasis on how the programming element will behave or will be accessed in the program.
1.Default: Identifies a property as the default property of its class, structure, or interface.
2.Module: Specifies that an attribute at the beginning of a source file applies to the current assembly module.
3.Private: Specifies that one or more declared programming elements are accessible only from within their declaration context, including from within any contained types.
4.Public: Specifies that one or more declared programming elements have no access restrictions.
5.ReadOnly: Specifies that a variable or property can be read but not written.
6.Shared: Specifies that one or more declared programming elements are associated with a class or structure at large, and not with a specific instance of the class or structure.
7.Static: Specifies that one or more declared local variables are to continue to exist and retain their latest values after termination of the procedure in which they are declared.
8.WriteOnly: Specifies that a property can be written but not read.
Statements: A statement is a complete instruction in vb programs. It may contain keywords, operators, variables etc.
1) Declaration statements : These are the statements where you name a variable, constant or procedure and can specify a data type.
2) Executable statements : These are the statements which initiate actions. These statements can call a method or function, loop or expression to a variable or constant.
Declaration statements:
1.Dim : Declares and allocates storage space for one of more variables.
2.Const : Declares and defines one or more constants.
3.Enum : Declares an enumeration and defines the values of its members.
4.Class : Declares the name of a class and introduces the definition of the variables, properties, events, and procedures that the class comprises.
5.Structure: Declares the name of a structure and introduces the definition of the variables, properties, events and procedures that the structure comprises.
6.Module: Declares the name of a module and introduces the definition of the variables, properties, events and procedures that the module comprises.
7.Sub: Declares the name, parameters and code that define a sub procedure.
8.Function: Declares the name, parameters and code that define a function procedure.
9.Operator: Declares the operator symbol, operands and code that define an operator procedure on a class or structure.
IF THEN ELSE:
syntax: If expression Then
statements
Else If
statements
Else
statements
End If
Operators: An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations.
1.Arithmetic Operators:
^ : raise one operand to the power of another
+ : adds two operands
- : subtract second operand from the first
* : multiplies both operands
/ : divides one operand by another and return a floating point result
\ : divides one operand by another and return a integer result
MOD : modulus operator and remainder of after an integer division
2.Comparison Operators:
= : check if the value of two operands are equal or not. if yes then condition becomes true.
<> : check if the values of two operands are equal or not. if not equal then condition becomes true.
> : check if the value of left operand is greater than the value of right operand.
< : check if the value of left operand is less than the value of the right operand.
>= : check if the value of left operand is greater than or equal to the value of the right operand.
<= : check if the value of left operand is less than or equal to the value of the right operand.
3.Logical or bitwise Operators:
And : It is the logical as well as bitwise AND operator. If both the operands are true, then condition becomes true.
Or : It is the logical as well as bitwise OR operator. If any of the two operands is true, then condition becomes true.
Not : It is the logical as well as bitwise NOT operator. Use to reverses the logical state of its operand.
If a condition is true, then logical NOT operator will make false.
Xor : It is the logical as well as bitwise logical exclusive OR operator.
It returns true if both expressions are true or both expressions are false. Otherwise it returns false.
OrElse : It is the logical OR operator. It works only on boolean data.
IsFalse : It determines whether an expression is False.
IsTrue : It determines whether an expression is True.
AndAlso : It is the logical AND operator. It works only on boolean data.
4.Assignment Operators:
= : Simple assignment operator, Assign values from right side operands to left side operand.
+= : Add AND assignment operator, It adds right operand to the left operand and assigns the result to left operand.
-= : Subtract AND assignment operator, It subtract right operand from the left operand and assigns the result to left operand.
*= : It multiplies right operand with the left operand and assign the result to left operand.
/= : It divides left operand with the right operand and assigns the result to left operand.(float)
\= : It divides left operand with the right operand and assigns the result to left operand.(int)
^= : It raises the left operand to the power of the right operand and assigns the result to left operand.
<<= : Left shift AND assignment operator.
>>= : Right shift AND assignment operator.
&= : Concatenates a string expression to a string variable or property and assigns the result to the variable or property.
Loops:
Do : syntax
Do { While | Until } condition Do
[ statements ] [ statements ]
[ Continue Do ] [ OR ] [ Continue Do ]
[ statements ] [ statements ]
[ Exit Do ] [ Exit Do ]
[ statements ] [ statements ]
Loop Loop { While | Until } condition
Ex:
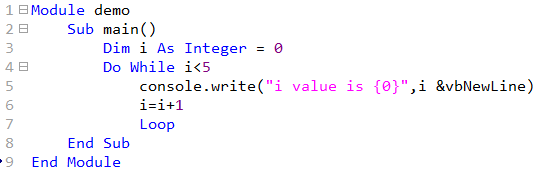
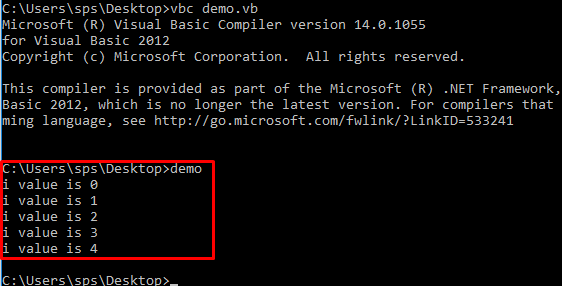
For Next: syntax
For counter [ As datatype ] = start To end [ Step step ]
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ counter ]
Ex:
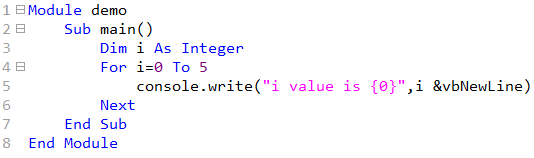
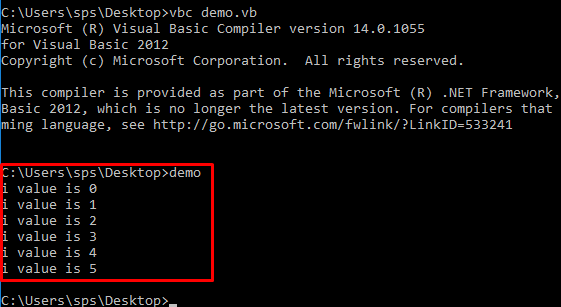
For Each Next: syntax
For Each element [ As datatype ] In group
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ element ]
Ex:
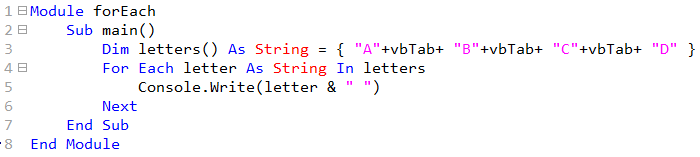
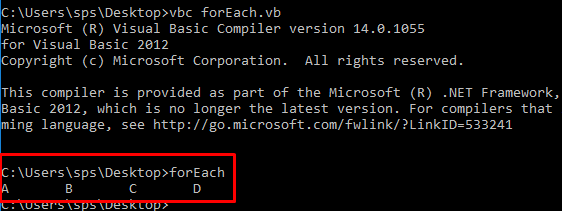
While End While: syntax
While condition
[ statements ]
[ Continue While ]
[ statements ]
[ Exit While ]
[ statements ]
End While
Ex:
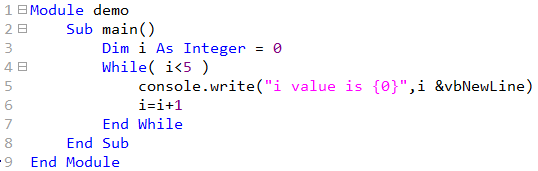
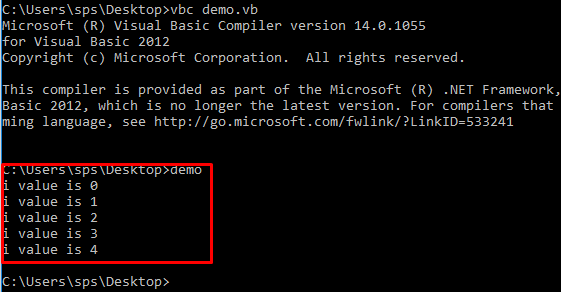
With End With: syntax
With object
[ statements ]
End With
Loop Control Statements:
Exit: Terminates the loop or select case statement and transfer execution to the statement immediately following the loop or select case.
Syntax:
Exit { Do | For | Function | Property | Select | Sub | Try | While }
Continue: Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating.
Syntax:
Continue { Do | For | While }
GoTo: Transfer control to the labeled statement. Though it is not advised to use GoTo statement in your program.
Syntax:
Label 1
[ statements ]
GoTo Lable1
[ statements ]
Arrays: An array stores a fixed size sequential collection of elements of the same type. All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element.
To declare an array in vb.net Dim statement is used.
Dim intData(10) an array of 11 elements
Dim strData(20) As String an array of 21 strings
Dim twoDarr(10,20) As String a two dimensional array of integers
To initialize the array elements while declaring.
Dim intData() As Integer = { 1, 2, 3, 4, 5, 6 }
Dim names() As String = { "Adam", "Ben", "Canny", "Dolly", "Enriq" }
Dim miscData() As Object = { "TurboModus", 500084, "Hyderabad" }
Ex:
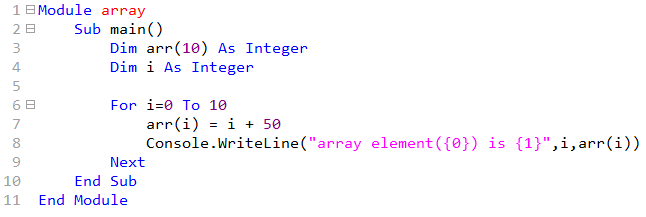
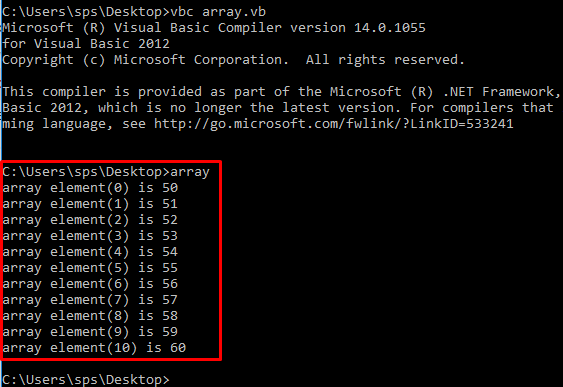
Dynamic Arrays: Dynamic arrays are arrays that can be dimensioned and re-dimensioned as per the need of the program. To declare a dynamic array ReDim statement is used.
Syntax: ReDim [ Preserve ] arrayname ( subscripts )
Where, Preserve keyword helps to preserve the data in an existing array, when resize it.
arrayname is the name of the array to re-dimension.
subscripts specifies the new dimension.
Ex:
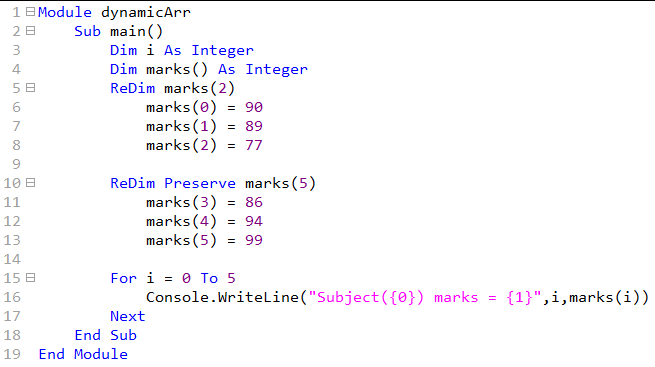
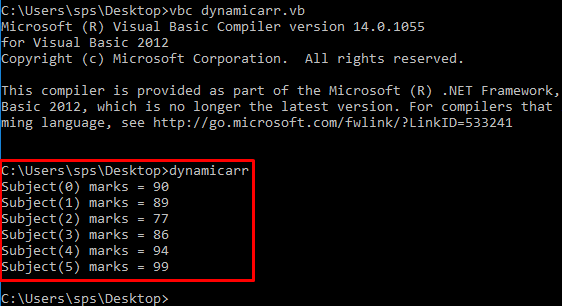
The Array Class: The Array class is the base class for all the arrays in vb.net. It is defined in the system namespace. The array class provide various properties to work with arrays.
Properties of array class:
IsFixedSizse: Gets a value indicating whether the array has a fixed size.
IsReadOnly: Gets a value indicating whether the array is read only.
Length: Gets a 32 bit integer that represents the total number of elements in all the dimensions of the array.
LongLength: Gets a 64 bit integer that represents the total number of elements in all the dimenstions of the array.
Rank: Gets the rank of the array. Here rank means number of dimensions.
Collections
Collection classes are specialized classes for data storage and retrieval. These classes provide support for stacks, queues, lists and hash tables. These classes create collections of objects of the object class, which is the base class for all data types in vb.net.
ArrayList: It represents ordered collection of an object that can be indexed individually. It is basically an alternative to an array. However, unlike array, you can add and remove items from a list at a specified position using an index and the array resizes itself automatically.
Hashtable: It uses a key to access the elements in the collection. A hash table is used when you need to access elements by using key, and you can identify a useful key value. Each item in the hash table has a key/value pair. The key is used to access the items in the collection.
SortedList: It uses a key as well as an index to access the items in a list. A sorted list is a combination of an array and a hash table. It contains a list of items that can be accessed using a key or an index. If you access items using an index, it is an ArrayList, and if you access items using a key, it is a Hashtable. The collection of items is always sorted by the key value.
Stack: It represents a last-in, first out collection of objects. It is used when you need a last-in, first-out access of items. When you add an item in the list, it is called pushing the item, and when you remove it, it is called popping the item.
Queue: It represents a first-in, first out collection of object. It is used when you need a first-in, first out access of items. When you add an item in the list, it is called enqueue, and when you remove an item it is called deque.
BitArray: It represents an array of the binary representation using the values 1 to 0. It is used when you need to store the bits but do not know the number of bits in advance. You can access items from the BitArray collection by using an integer index, which starts from zero.
Functions:
Syntax: Dim varName As varType
Here varName is the name of the variable.
varType is the datatype of the variable.
Data type: Data type define the type of data that a variable can store.
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------
VB alias .Net Type Size Range
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------
SByte System.SByte 8 bits -128 to 127
Byte System.Byte 8 bits 0 to 255
Short System. Int16 16 bits -32,768 to 32,767
UShort System.UInt16 16 bits 0 to 65,535
Integer System.Int32 32 bits -2147483648 to 2147483647
UInteger System.UInt32 32 bits 0 to 4294967295
Long System.Int64 64 bits -9223372036854775808 to 9223372036854775807
ULong System.UInt64 64 bits 0 to 18446744073709551615
Single System.Single 32 bits
Double System.Double 64 bits
Decimal System.Decimal 128 bits
Char System.Char 16 bits one unicode symbol in the range of 0 to 65535
Boolean System.Boolean 32 bits true or false
Object System.Object 32/64 bits platform dependent
Date System.DateTime 64 bits Jan 1,0001 12:00:00 am to Dec 31,9999 11:59:59 pm
String System.String 80+[16xLength]bits
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Variable Initialization: Variables are initialized with an equal sign followed by a constant expression. The general form of initialization is.
ex: Dim pi As Double
pi=3.14159
Accepting values from user: The Console class in the system namespace provides a function ReadLine for accepting input from the user and store it into a variable.
ex: Dim message As String
message = Console.ReadLine
Declaring Constants: Constants are declared using the Const statement. The const statement is used at module, class, structure, procedure or block level for use in place of literal values.
syntax: constantname [ As datatype ] = initializer
Print and Display constants:
vbNewLine : new line character.
vbNullChar : null character.
vbNullString : not the same as a zero length stirng, used for calling external procedures.
vbTab : tab character.
vbBack : backspace character.
vbCrLf : carriage return/linefeed character combination.
vbCr : carriage return character.
vbLf : Linefeed character.
Modifiers: The modifiers are keywords added with any programming element to give some especial emphasis on how the programming element will behave or will be accessed in the program.
1.Default: Identifies a property as the default property of its class, structure, or interface.
2.Module: Specifies that an attribute at the beginning of a source file applies to the current assembly module.
3.Private: Specifies that one or more declared programming elements are accessible only from within their declaration context, including from within any contained types.
4.Public: Specifies that one or more declared programming elements have no access restrictions.
5.ReadOnly: Specifies that a variable or property can be read but not written.
6.Shared: Specifies that one or more declared programming elements are associated with a class or structure at large, and not with a specific instance of the class or structure.
7.Static: Specifies that one or more declared local variables are to continue to exist and retain their latest values after termination of the procedure in which they are declared.
8.WriteOnly: Specifies that a property can be written but not read.
Statements: A statement is a complete instruction in vb programs. It may contain keywords, operators, variables etc.
1) Declaration statements : These are the statements where you name a variable, constant or procedure and can specify a data type.
2) Executable statements : These are the statements which initiate actions. These statements can call a method or function, loop or expression to a variable or constant.
Declaration statements:
1.Dim : Declares and allocates storage space for one of more variables.
2.Const : Declares and defines one or more constants.
3.Enum : Declares an enumeration and defines the values of its members.
4.Class : Declares the name of a class and introduces the definition of the variables, properties, events, and procedures that the class comprises.
5.Structure: Declares the name of a structure and introduces the definition of the variables, properties, events and procedures that the structure comprises.
6.Module: Declares the name of a module and introduces the definition of the variables, properties, events and procedures that the module comprises.
7.Sub: Declares the name, parameters and code that define a sub procedure.
8.Function: Declares the name, parameters and code that define a function procedure.
9.Operator: Declares the operator symbol, operands and code that define an operator procedure on a class or structure.
IF THEN ELSE:
syntax: If expression Then
statements
Else If
statements
Else
statements
End If
Operators: An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations.
1.Arithmetic Operators:
^ : raise one operand to the power of another
+ : adds two operands
- : subtract second operand from the first
* : multiplies both operands
/ : divides one operand by another and return a floating point result
\ : divides one operand by another and return a integer result
MOD : modulus operator and remainder of after an integer division
2.Comparison Operators:
= : check if the value of two operands are equal or not. if yes then condition becomes true.
<> : check if the values of two operands are equal or not. if not equal then condition becomes true.
> : check if the value of left operand is greater than the value of right operand.
< : check if the value of left operand is less than the value of the right operand.
>= : check if the value of left operand is greater than or equal to the value of the right operand.
<= : check if the value of left operand is less than or equal to the value of the right operand.
3.Logical or bitwise Operators:
And : It is the logical as well as bitwise AND operator. If both the operands are true, then condition becomes true.
Or : It is the logical as well as bitwise OR operator. If any of the two operands is true, then condition becomes true.
Not : It is the logical as well as bitwise NOT operator. Use to reverses the logical state of its operand.
If a condition is true, then logical NOT operator will make false.
Xor : It is the logical as well as bitwise logical exclusive OR operator.
It returns true if both expressions are true or both expressions are false. Otherwise it returns false.
OrElse : It is the logical OR operator. It works only on boolean data.
IsFalse : It determines whether an expression is False.
IsTrue : It determines whether an expression is True.
AndAlso : It is the logical AND operator. It works only on boolean data.
4.Assignment Operators:
= : Simple assignment operator, Assign values from right side operands to left side operand.
+= : Add AND assignment operator, It adds right operand to the left operand and assigns the result to left operand.
-= : Subtract AND assignment operator, It subtract right operand from the left operand and assigns the result to left operand.
*= : It multiplies right operand with the left operand and assign the result to left operand.
/= : It divides left operand with the right operand and assigns the result to left operand.(float)
\= : It divides left operand with the right operand and assigns the result to left operand.(int)
^= : It raises the left operand to the power of the right operand and assigns the result to left operand.
<<= : Left shift AND assignment operator.
>>= : Right shift AND assignment operator.
&= : Concatenates a string expression to a string variable or property and assigns the result to the variable or property.
Loops:
Do : syntax
Do { While | Until } condition Do
[ statements ] [ statements ]
[ Continue Do ] [ OR ] [ Continue Do ]
[ statements ] [ statements ]
[ Exit Do ] [ Exit Do ]
[ statements ] [ statements ]
Loop Loop { While | Until } condition
Ex:
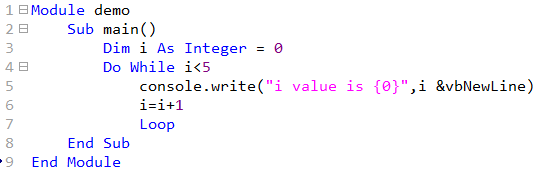
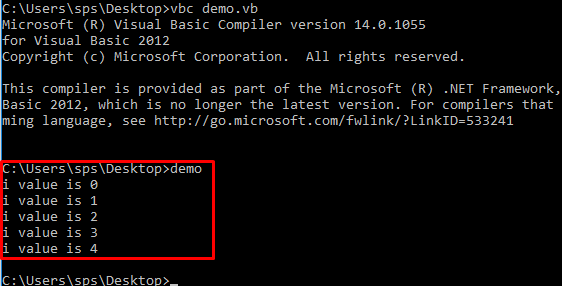
For Next: syntax
For counter [ As datatype ] = start To end [ Step step ]
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ counter ]
Ex:
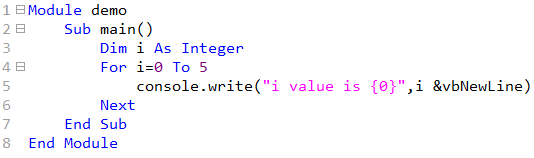
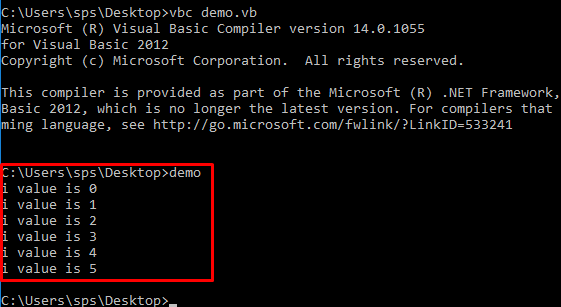
For Each Next: syntax
For Each element [ As datatype ] In group
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ element ]
Ex:
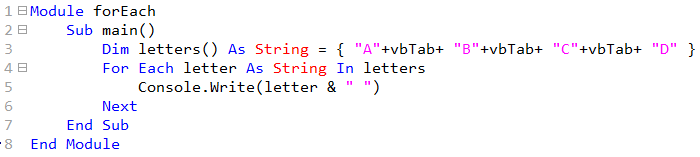
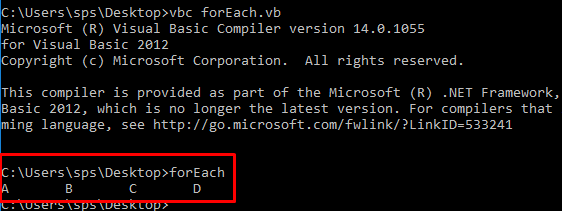
While End While: syntax
While condition
[ statements ]
[ Continue While ]
[ statements ]
[ Exit While ]
[ statements ]
End While
Ex:
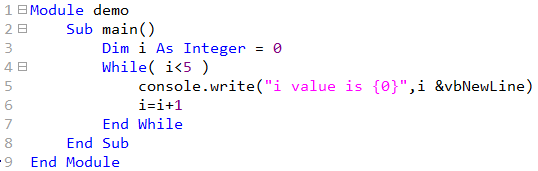
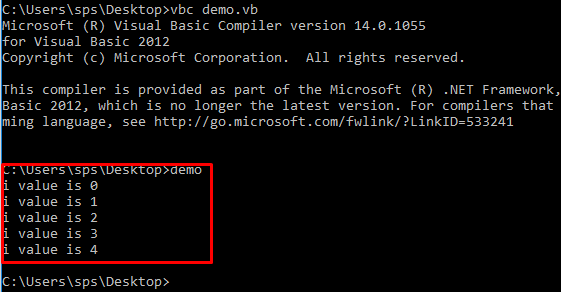
With End With: syntax
With object
[ statements ]
End With
Loop Control Statements:
Exit: Terminates the loop or select case statement and transfer execution to the statement immediately following the loop or select case.
Syntax:
Exit { Do | For | Function | Property | Select | Sub | Try | While }
Continue: Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating.
Syntax:
Continue { Do | For | While }
GoTo: Transfer control to the labeled statement. Though it is not advised to use GoTo statement in your program.
Syntax:
Label 1
[ statements ]
GoTo Lable1
[ statements ]
Arrays: An array stores a fixed size sequential collection of elements of the same type. All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element.
To declare an array in vb.net Dim statement is used.
Dim intData(10) an array of 11 elements
Dim strData(20) As String an array of 21 strings
Dim twoDarr(10,20) As String a two dimensional array of integers
To initialize the array elements while declaring.
Dim intData() As Integer = { 1, 2, 3, 4, 5, 6 }
Dim names() As String = { "Adam", "Ben", "Canny", "Dolly", "Enriq" }
Dim miscData() As Object = { "TurboModus", 500084, "Hyderabad" }
Ex:
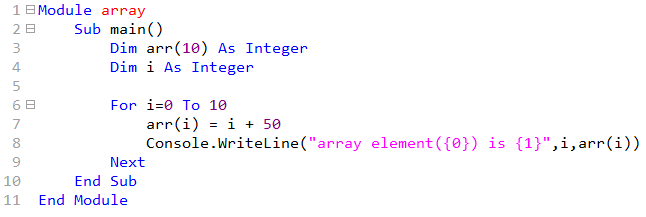
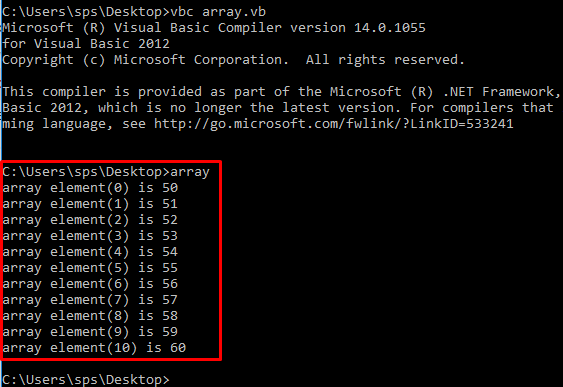
Dynamic Arrays: Dynamic arrays are arrays that can be dimensioned and re-dimensioned as per the need of the program. To declare a dynamic array ReDim statement is used.
Syntax: ReDim [ Preserve ] arrayname ( subscripts )
Where, Preserve keyword helps to preserve the data in an existing array, when resize it.
arrayname is the name of the array to re-dimension.
subscripts specifies the new dimension.
Ex:
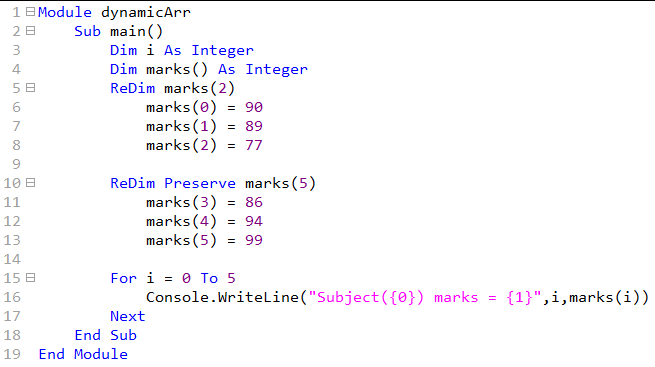
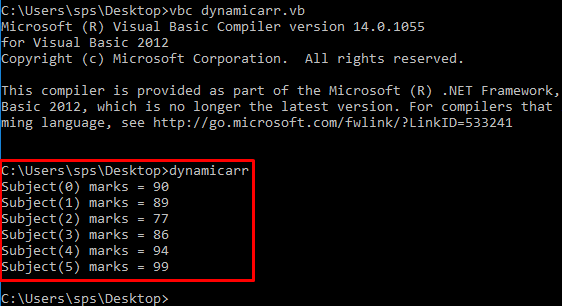
The Array Class: The Array class is the base class for all the arrays in vb.net. It is defined in the system namespace. The array class provide various properties to work with arrays.
Properties of array class:
IsFixedSizse: Gets a value indicating whether the array has a fixed size.
IsReadOnly: Gets a value indicating whether the array is read only.
Length: Gets a 32 bit integer that represents the total number of elements in all the dimensions of the array.
LongLength: Gets a 64 bit integer that represents the total number of elements in all the dimenstions of the array.
Rank: Gets the rank of the array. Here rank means number of dimensions.
Collections
Collection classes are specialized classes for data storage and retrieval. These classes provide support for stacks, queues, lists and hash tables. These classes create collections of objects of the object class, which is the base class for all data types in vb.net.
ArrayList: It represents ordered collection of an object that can be indexed individually. It is basically an alternative to an array. However, unlike array, you can add and remove items from a list at a specified position using an index and the array resizes itself automatically.
Hashtable: It uses a key to access the elements in the collection. A hash table is used when you need to access elements by using key, and you can identify a useful key value. Each item in the hash table has a key/value pair. The key is used to access the items in the collection.
SortedList: It uses a key as well as an index to access the items in a list. A sorted list is a combination of an array and a hash table. It contains a list of items that can be accessed using a key or an index. If you access items using an index, it is an ArrayList, and if you access items using a key, it is a Hashtable. The collection of items is always sorted by the key value.
Stack: It represents a last-in, first out collection of objects. It is used when you need a last-in, first-out access of items. When you add an item in the list, it is called pushing the item, and when you remove it, it is called popping the item.
Queue: It represents a first-in, first out collection of object. It is used when you need a first-in, first out access of items. When you add an item in the list, it is called enqueue, and when you remove an item it is called deque.
BitArray: It represents an array of the binary representation using the values 1 to 0. It is used when you need to store the bits but do not know the number of bits in advance. You can access items from the BitArray collection by using an integer index, which starts from zero.
Functions: