Definition: A function is a set of statements that take inputs, do some specific computation and produces output. The idea is to put some commonly or repeatedly done task together and make a function, so that instead of writing the same code again and again for different inputs, we can call the function.
Ex: int max(int x, int y)
{
if( x>y)
return x;
else
return y;
}
Parts of a function:
Return type: A function may return a value. The return type is the data type of the value the function returns. Some functions perform the desired operations without returning a value. In this case the return type is void.
Function name: This is the actual name of the function. The function name and the parameter list together constitute the function signature.
Parameters: When a function is invoked, you pass a value to the parameter. This value is referred to as actual parameter or argument. The parameter list refers to the type, order, and number of the parameters of a function. Parameters are optional.
Function body: The function body contains a collection of statements that define what the function does.
Declaration: Function declaration tells compiler about number of parameters function takes, data types of parameters and return type of function. Putting parameter names in function declaration is optional, but it is necessary to put them in definition.
In the above example,
int is return type
max is function name
x,y are parameters
with in {} is body.
Function Calling: When a program calls a function, the program control is transferred to the called function. A called function performs a defined task and when its return statement is executed or when its function ending closing brace is reached, it returns the program control back to the main program. To call a function, you simply need to pass the required parameters along with the function name, and if the function returns a value, then you can store the returned value.
Ex: MaxNum=max(a,b);
System Defined: The standard library functions are built in functions in C programming to handle tasks such as mathematical computations, i/o processing, string handling etc.
These functions are defined in the header file. When you include the header file, these functions are available for use.
Ex: The printf() is a standard library function to send formatted output to the screen. This function is defined in stdio.h header file.
User Defined: Which functions are defined by the user at the time of writing program is called user defined functions. These functions are made for code reusability and for saving time and space.
Ex: int add(int x, int y)
{
int sum;
sum=x+y;
return sum;
}
Input/output function: These functions are used to read input from keyboard and write output on display.
1. printf(): It is used to display output on screen.
2. scanf(): It is used to take input from keyboard.
3. getchar(): It reads a single character at a time from the terminal.
4. putchar(): It display the character passed to it on the screen and returns the same character.
5. gets(): It reads a line from stdin into the buffer pointed to by str pointer, until either a terminating newline or EOF occurs.
6. puts(): It writes the string str and a trailing newline to stdout.
Console io function: Keyboard and screen together called console. These functions receive input from keyboard and write them on the display.
1. getch(): Get a character from the keyboard.
2. getche(): Get a character from the keyboard and echo it.
File io function: These functions perform input/output operations on a floppy or hard disk.
Mathematical function: These functions are used to perform mathematical operations. Math.h header file supports all the mathematical related functions.
1. floor(): This function returns the nearest integer which is less than or equal to the argument passed to this function.
2. ceil(): This function returns nearest integer value which is greater than or equal to the argument passed to this function.
3. pow(): This is used to find the power of the given number.
4. sqrt(): This function is used to find square root of the argument passed to this function.
Write a function to add two numbers.
Code:
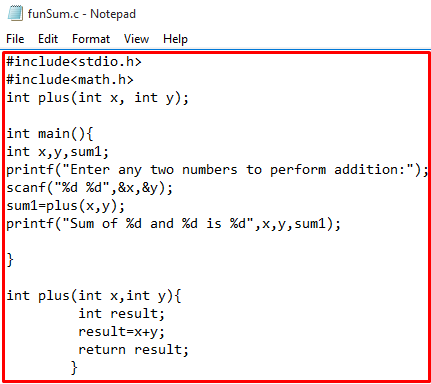
Compile & Run:
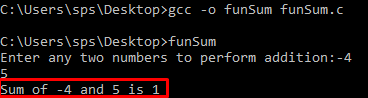
Write a function to display multiplication table.
Code:
Compile & Run:
Ex: int max(int x, int y)
{
if( x>y)
return x;
else
return y;
}
Parts of a function:
Return type: A function may return a value. The return type is the data type of the value the function returns. Some functions perform the desired operations without returning a value. In this case the return type is void.
Function name: This is the actual name of the function. The function name and the parameter list together constitute the function signature.
Parameters: When a function is invoked, you pass a value to the parameter. This value is referred to as actual parameter or argument. The parameter list refers to the type, order, and number of the parameters of a function. Parameters are optional.
Function body: The function body contains a collection of statements that define what the function does.
Declaration: Function declaration tells compiler about number of parameters function takes, data types of parameters and return type of function. Putting parameter names in function declaration is optional, but it is necessary to put them in definition.
In the above example,
int is return type
max is function name
x,y are parameters
with in {} is body.
Function Calling: When a program calls a function, the program control is transferred to the called function. A called function performs a defined task and when its return statement is executed or when its function ending closing brace is reached, it returns the program control back to the main program. To call a function, you simply need to pass the required parameters along with the function name, and if the function returns a value, then you can store the returned value.
Ex: MaxNum=max(a,b);
System Defined: The standard library functions are built in functions in C programming to handle tasks such as mathematical computations, i/o processing, string handling etc.
These functions are defined in the header file. When you include the header file, these functions are available for use.
Ex: The printf() is a standard library function to send formatted output to the screen. This function is defined in stdio.h header file.
User Defined: Which functions are defined by the user at the time of writing program is called user defined functions. These functions are made for code reusability and for saving time and space.
Ex: int add(int x, int y)
{
int sum;
sum=x+y;
return sum;
}
Input/output function: These functions are used to read input from keyboard and write output on display.
1. printf(): It is used to display output on screen.
2. scanf(): It is used to take input from keyboard.
3. getchar(): It reads a single character at a time from the terminal.
4. putchar(): It display the character passed to it on the screen and returns the same character.
5. gets(): It reads a line from stdin into the buffer pointed to by str pointer, until either a terminating newline or EOF occurs.
6. puts(): It writes the string str and a trailing newline to stdout.
Console io function: Keyboard and screen together called console. These functions receive input from keyboard and write them on the display.
1. getch(): Get a character from the keyboard.
2. getche(): Get a character from the keyboard and echo it.
File io function: These functions perform input/output operations on a floppy or hard disk.
Mathematical function: These functions are used to perform mathematical operations. Math.h header file supports all the mathematical related functions.
1. floor(): This function returns the nearest integer which is less than or equal to the argument passed to this function.
2. ceil(): This function returns nearest integer value which is greater than or equal to the argument passed to this function.
3. pow(): This is used to find the power of the given number.
4. sqrt(): This function is used to find square root of the argument passed to this function.
Write a function to add two numbers.
Code:
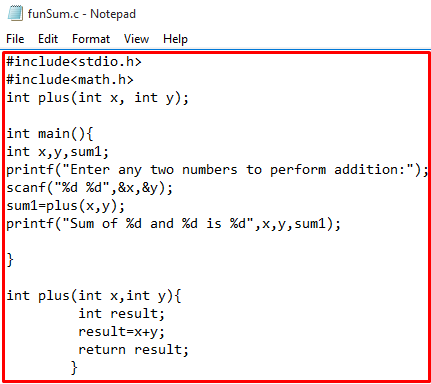
Compile & Run:
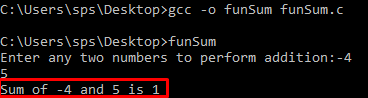
Write a function to display multiplication table.
Code:
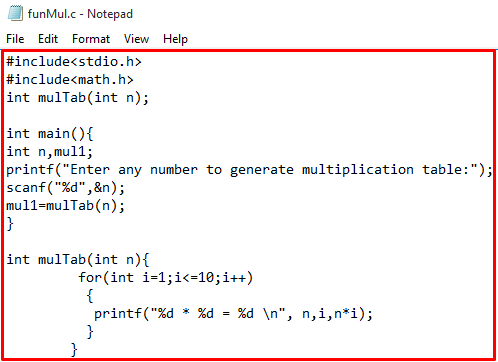
Compile & Run:
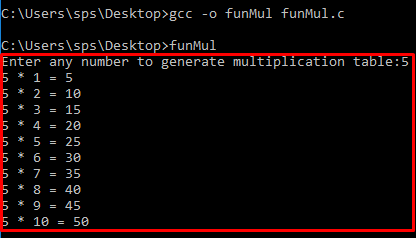