IF: The if statement evaluates the test expression inside the parenthesis.
Syntax: if(TestExpression)
{
Statements;
}
IF...ELSE: If test expression is evaluated to true,
If test expression is evaluated to false,
- If the test expression is evaluated to true, statements inside the body of if is executed.
- If the test expression is evaluated to false, statements inside the body of if is skipped from execution.
Syntax: if(TestExpression)
{
Statements;
}
IF...ELSE: If test expression is evaluated to true,
- statements inside the body of if statement is executed.
- statements inside the body of else statement is skipped from execution.
If test expression is evaluated to false,
- statements inside the body of else statement is executed.
- statements inside the body of if statement is skipped from execution
Syntax: if( Expression)
{
Statement;
}
else
{
Statement;
}
Nested IF: It is possible to include if statements inside the body of another if statement. When a series of decision are involved in statement, we use if statement in nested form.
Syntax: if( Expression)
{
if( Expression)
{
Statement;
}
}
Switch: Switch statement acts as a substitute for long if-else-if ladder that is used to test a list of cases. A switch statement contains one or more case labels which are tested against the switch expression. When the expression match to a case then the associated statements with that case would be executed.
Syntax: switch( Expression)
{
case value1:
Statements;
break;
case value2:
Statements;
break;
case value3:
Statements;
break;
case value n:
Statements;
break;
default:
Statements;
}
Ask the user to enter 3 numbers and display the largest number in that 3 numbers.
Code:
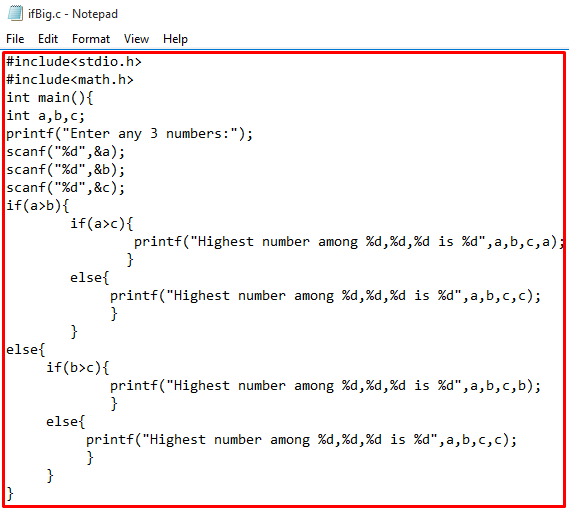
Compile & Run:
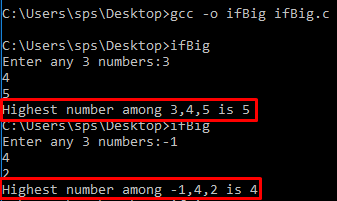
Looping Statements: When you need to execute a block of code several number of times then you need to use looping concept. A loop statement allows us to execute a statement or group of statements multiple times.
For: For loop is a statement which allows code to be repeatedly executed. For loop contains 3 parts initialization, condition and increment or decrements.
Syntax: for( initialization; condition; increment/decrement)
{
loop body;
}
While: In while loop first check the condition if condition is true then control goes inside the loop body other wise goes outside the body.
Syntax: While( condition)
{
loop body;
increment/decrement;
}
Do...While: A do..while loop is similar to a while loop, except that a do..while loop is execute at least one time. A do..while loop is a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given condition at the end of the block.
Syntax: do
{
Statements;
increment/decrement;
}while();
Break to jump out of the loop: When a break statement is encountered inside a loop, the loop is immediately terminated and the program control resumes at the next statement following the loop.
Syntax: break;
Continue: The continue statement skips statements after it inside the loop.
Syntax: continue;
Ask the user to enter a number and display the multiplication table of that number.
Code:
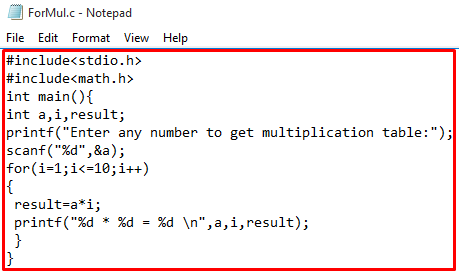
Compile & Run:
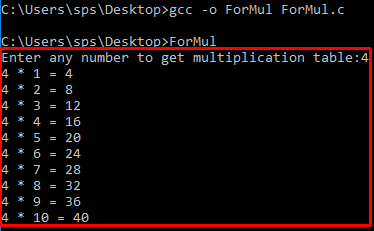
Code:
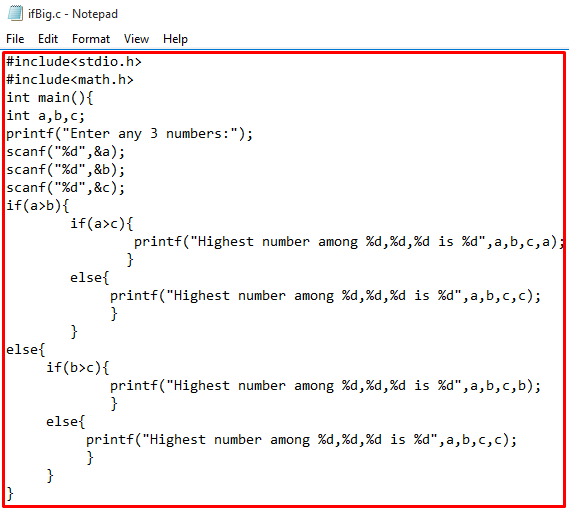
Compile & Run:
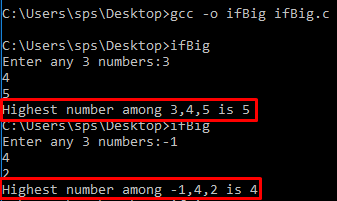
Looping Statements: When you need to execute a block of code several number of times then you need to use looping concept. A loop statement allows us to execute a statement or group of statements multiple times.
For: For loop is a statement which allows code to be repeatedly executed. For loop contains 3 parts initialization, condition and increment or decrements.
Syntax: for( initialization; condition; increment/decrement)
{
loop body;
}
While: In while loop first check the condition if condition is true then control goes inside the loop body other wise goes outside the body.
Syntax: While( condition)
{
loop body;
increment/decrement;
}
Do...While: A do..while loop is similar to a while loop, except that a do..while loop is execute at least one time. A do..while loop is a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given condition at the end of the block.
Syntax: do
{
Statements;
increment/decrement;
}while();
Break to jump out of the loop: When a break statement is encountered inside a loop, the loop is immediately terminated and the program control resumes at the next statement following the loop.
Syntax: break;
Continue: The continue statement skips statements after it inside the loop.
Syntax: continue;
Ask the user to enter a number and display the multiplication table of that number.
Code:
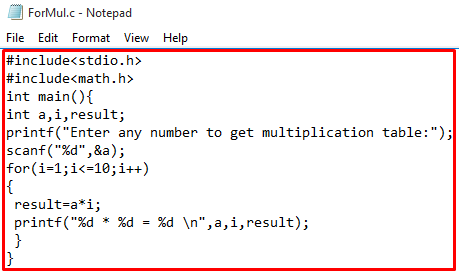
Compile & Run:
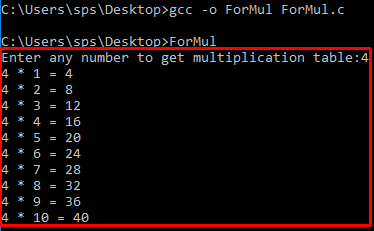