C++ is an extension to C language and it is an intermediate level language. C++ is an Object Oriented programming language but it is not purely Object Oriented.
Benefits:
1. C++ is an object oriented language whereas C is a procedural language.
2. There is strong type checking in C++.
3. C++ supports and allows user defined operators and function overloading is also supported in it.
4. Exception handling is there in C++.
Object Oriented features:
Object: Object is the physical as well as logical entity where as class is the only logical entity. Objects are instances of class, which have data members and uses various member functions to perform tasks.
Class: Class is a blue print which is containing only list of variables and method and no memory is allocated for them. A class is a group of objects that has common properties.
1. Abstraction: Data abstraction refers to, providing only essential information to the outside world and hiding their background details, i.e., to present the needed information in program without presenting the details.
2. Encapsulation: Encapsulation is placing the data and the functions that work on that data in the same place. While working with procedural languages, it is not always clear which functions work on which variables but object oriented programming provides you framework to place the data and the relevant functions together in the same object.
3. Inheritance: Inheritance is the process of forming a new class from an existing class that is from the existing class called as base class, new class is formed called as derived class. It helps to reduce the code size.
4. Polymorphism: The ability to use an operator or function in different ways is called polymorphism.
Write simple Hello world program.
Code:
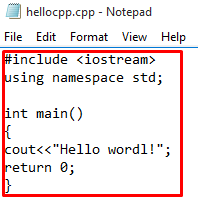
Compile & Run:
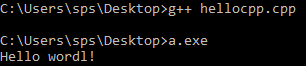
Variables: A variable is a name which is associated with a value that can be changed.
Syntax: datatype variableName=value;
Ex: int num=10;
Types of variables: Any variable declared inside the curly braces have scope limited these curly braces, if you declare a variable in main() function and try to use that variable outside main() function then you will get compilation error.
1. Local variable: Local variables are declared inside the braces of any user defined function, main function, loops or any control statements and have their scope limited inside those braces.
2. Global variable: A variable declared outside of any function is called global variable. Global variables have their scope throughout the program, they can be accessed anywhere in the program, in the main, in the user defined function, anywhere.
Write a code to display local and global values.
Code:
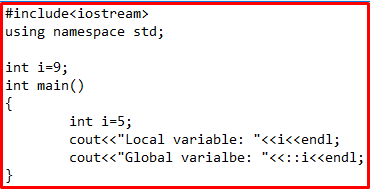
Compile & Run:
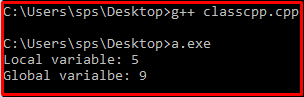
Data types: Data types define the type of data a variable can hold, For example an integer variable can hold integer data, a character type variable can hold character data.
Data types in c++ are categorised in three groups: Built-in, User-defined and Derived.
Built in:
1. Char 1 byte -127 to 127 or 0 to 255
2. Int 4 bytes 0 to 255
3. Float 4 bytes
4. Double 8 bytes
5. Bool 1 byte
6. Wchar_t 2 or 4 bytes 1 wide character
User defined:
1. Struct: A structure is a user defined data type. A structure creates a data type that can be used to group items of possibly different types into a single type.
Ex: Struct address{
char name[50];
char street[100];
char city[50];
char state[50];
int pin;
2. Union: In union, all members share the same memory location.
3. Enum: Enumeration is a user defined data type. It is mainly used to assign names to integral constants, the names make a program easy to read and maintain.
Syntax: enum stae{working=1, failed=0};
Ex: enum week { Mon, Tue, Wed, Thu, Fri, Sat, Sun};
Derived: Data types that are derived from the built in data types are known as derived data types.
1. Array: An array is a set of elements of the same data type that are referred to by the same name. All elements in array are stored at contiguous memory locations and each element is accessed by a unique index.
2. Function: A function is a self contained program segment that carries out a specific well defined task.
3. Pointer: A pointer is a variable that can store the memory address of another variable. Pointers allow to use the memory dynamically.
Data type modifiers: Data type modifiers are used with the built in data types to modify the length of data that a particular data type to modify the length of data that a particular data type can hold.
1. Signed: Signed types includes both positive and negative numbers and is the default type.
2. Unsigned: Unsigned numbers are always without any sign, that is always positive.
3. Short: Modify the minimum values that a data type will hold.
4. Long: Modify the maximum values that a data type will hold.
Operators: Operator represents an action. For example, + is an operator that represent addition. An operator works on two or more operands and produce an output.
Types:
1. Arithmetic Operators:
+ is for addition.
- is for subtraction.
* is for multiplication
/ is for division.
% is for modulo.
2. Assignment Operators:
=, +=, -=, *=, /=, %=
3. Auto-increment and auto-decrement operators:
++ and - -
4. Logical operators:
&&, ||, !
5. Relational operators:
==, !=, >, <, >=, <=
6. Bitwise operators:
&, |, ^, ~, <<, >>
Conditional Statements:
IF: The if statement evaluates the test expression inside the parenthesis.
Syntax: if(TestExpression)
{
Statements;
}
IF...ELSE: If test expression is evaluated to true,
If test expression is evaluated to false,
Conditional operator: The conditional operator returns one of two values depending on the result of an expression.
Syntax: (expression 1) ? expression 2 : expression 3
If expression 1 is evaluated to true, then expression 2 is evaluated.
If expression 1 is evaluated to false, then expression 3 is evaluated.
Looping Statements:
For: For loop is a statement which allows code to be repeatedly executed. For loop contains 3 parts initialization, condition and increment or decrements.
Syntax: for( initialization; condition; increment/decrement)
{
loop body;
}
While: In while loop first check the condition if condition is true then control goes inside the loop body other wise goes outside the body.
Syntax: While( condition)
{
loop body;
increment/decrement;
}
Do...While: A do..while loop is similar to a while loop, except that a do..while loop is execute at least one time. A do..while loop is a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given condition at the end of the block.
Syntax: do
{
Statements;
increment/decrement;
}while();
Nested loop: A loop can be nested inside of another loop. C++ allows at least 256 levels of nesting.
Syntax: The syntax for a nested for loop.
for( init; condition; increment){
for( init; condition; increment){
statements;
}
statements;
}
The syntax for a nested while loop.
while( condition) {
while( condition) {
statements;
}
statements;
}
The syntax for a nested do..while loop.
do {
statements;
do {
statements;
}while( condition);
}while( condition);
Loop control statements:
Break: When a break statement is encountered inside a loop, the loop is immediately terminated and the program control resumes at the next statement following the loop.
Syntax: break;
Continue: The continue statement skips statements after it inside the loop.
Syntax: continue;
Go to: Go to statement is used for altering the normal sequence of program execution by transferring control to some other part of the program. The go to statement can be used to jump from anywhere to anywhere within a function.
Syntax: goto label1;
..........................
..........................
label1:
statements;
...........................
Functions: A function is a group of statements that together perform a task. A function declaration tells the compiler about a function's name, return type, and parameters. A function definition provides the actual body of the function.
There are two types of functions:
1. Library functions: Library functions are the built-in function, programmer can use library function by invoking function directly.
Ex: log(), pow(), sqrt(), abs() etc.
2. User defined functions: C++ allows programmer to define their own function. A user defined function groups code to perform a specific task and that group of code is given a name.
Write a code to display your name with spaces and without.
Code
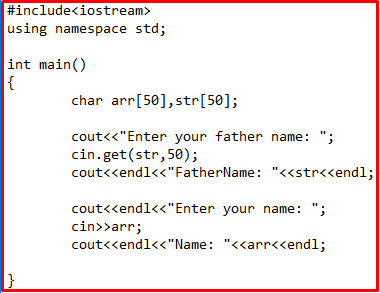
Compile & Run:
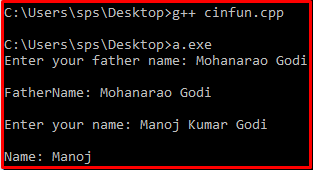
Recursion: The process in which a function calls itself is known as recursion and the corresponding function is called the recursive function.
Ex: factorial function.
Direct recursion: When function calls itself, it is called direct recursion.
Indirect recursion: When function calls another function and that function calls the calling function, then this is called indirect recursion.
Arrays: An array is a series of elements of the same type placed in contiguous memory locations that can be individually referenced by adding an index to a unique identifier.
Why do we need arrays?
We can use normal variables(v1, v2,....vn) when we have small number of objects, but if we want to store large number of instances, it become difficult to manage them with normal variables. The idea of array is to represent many instances in one variable.
Declaration:
1. Declaration by specifying size
Ex: int arr[10];
2. Declaration by initializing elements
Ex: int arr[]={10,20,30}
3. Declaration by specifying size and initialising elements
Ex: int arr[5]={10,20,30,40}
Files:
The iostream standard library provides cin and cout methods for reading from standard input and writing to standard output. To read and write form a file requires another standared C++ library called fstream. Which defines three new data types.
Datatypes:
Ofstream: This data type represents the output file stream and is used to create files and to write information to files.
ifstream: Thsi data type represents the input file stream and is used to read information from files.
fstream: This datatype represents the file stream generally, and has the capabilities of both ofstream and ifstream which means it can create files, write information to files, and read information from files.
Note: To perform file processing in C++, header files <iostream> and <fstream> must be included.
Opening a file: A file must be opened before perform read or write operation on it. Either ofstream or fstream object may be used to open a file for writing. And ifstream object is used to open a file for reading purpose only.
Mode flag:
ios::app : Append mode. All output to that file to be appended to the end.
ios::ate : Open a file for output and move the read/write control to the end of the file.
ios::in : Open a file for reading.
ios::out : Open a file for writing.
ios::trunc : If the file already exists, its contents will be truncated before opening the file.
Closing a file: When a C++ program terminates it automatically flushes all the streams, release all the allocated memory and close all the opened files. But it is always a good practice that should close all the opened files before program termination.
Writing to a file: While doing c++ programming, you write information to a file from your program using the stream insertion operator just as you use that operator to output information to the screen. The only difference is that you use an ofstream or fstream object instead of the cout object.
Reading from a file: You read information from a file into your program using the stream extraction operator just as you use that operator to input information from the keyboard. The only difference is that you use an ifstream or fstream object instead of the cin object.
Benefits:
1. C++ is an object oriented language whereas C is a procedural language.
2. There is strong type checking in C++.
3. C++ supports and allows user defined operators and function overloading is also supported in it.
4. Exception handling is there in C++.
Object Oriented features:
Object: Object is the physical as well as logical entity where as class is the only logical entity. Objects are instances of class, which have data members and uses various member functions to perform tasks.
Class: Class is a blue print which is containing only list of variables and method and no memory is allocated for them. A class is a group of objects that has common properties.
1. Abstraction: Data abstraction refers to, providing only essential information to the outside world and hiding their background details, i.e., to present the needed information in program without presenting the details.
2. Encapsulation: Encapsulation is placing the data and the functions that work on that data in the same place. While working with procedural languages, it is not always clear which functions work on which variables but object oriented programming provides you framework to place the data and the relevant functions together in the same object.
3. Inheritance: Inheritance is the process of forming a new class from an existing class that is from the existing class called as base class, new class is formed called as derived class. It helps to reduce the code size.
4. Polymorphism: The ability to use an operator or function in different ways is called polymorphism.
Write simple Hello world program.
Code:
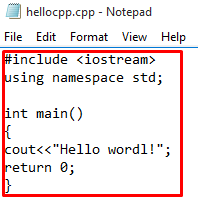
Compile & Run:
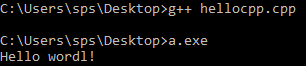
Variables: A variable is a name which is associated with a value that can be changed.
Syntax: datatype variableName=value;
Ex: int num=10;
Types of variables: Any variable declared inside the curly braces have scope limited these curly braces, if you declare a variable in main() function and try to use that variable outside main() function then you will get compilation error.
1. Local variable: Local variables are declared inside the braces of any user defined function, main function, loops or any control statements and have their scope limited inside those braces.
2. Global variable: A variable declared outside of any function is called global variable. Global variables have their scope throughout the program, they can be accessed anywhere in the program, in the main, in the user defined function, anywhere.
Write a code to display local and global values.
Code:
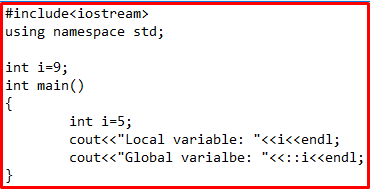
Compile & Run:
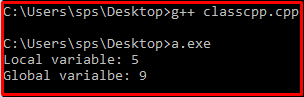
Data types: Data types define the type of data a variable can hold, For example an integer variable can hold integer data, a character type variable can hold character data.
Data types in c++ are categorised in three groups: Built-in, User-defined and Derived.
Built in:
1. Char 1 byte -127 to 127 or 0 to 255
2. Int 4 bytes 0 to 255
3. Float 4 bytes
4. Double 8 bytes
5. Bool 1 byte
6. Wchar_t 2 or 4 bytes 1 wide character
User defined:
1. Struct: A structure is a user defined data type. A structure creates a data type that can be used to group items of possibly different types into a single type.
Ex: Struct address{
char name[50];
char street[100];
char city[50];
char state[50];
int pin;
2. Union: In union, all members share the same memory location.
3. Enum: Enumeration is a user defined data type. It is mainly used to assign names to integral constants, the names make a program easy to read and maintain.
Syntax: enum stae{working=1, failed=0};
Ex: enum week { Mon, Tue, Wed, Thu, Fri, Sat, Sun};
Derived: Data types that are derived from the built in data types are known as derived data types.
1. Array: An array is a set of elements of the same data type that are referred to by the same name. All elements in array are stored at contiguous memory locations and each element is accessed by a unique index.
2. Function: A function is a self contained program segment that carries out a specific well defined task.
3. Pointer: A pointer is a variable that can store the memory address of another variable. Pointers allow to use the memory dynamically.
Data type modifiers: Data type modifiers are used with the built in data types to modify the length of data that a particular data type to modify the length of data that a particular data type can hold.
1. Signed: Signed types includes both positive and negative numbers and is the default type.
2. Unsigned: Unsigned numbers are always without any sign, that is always positive.
3. Short: Modify the minimum values that a data type will hold.
4. Long: Modify the maximum values that a data type will hold.
Operators: Operator represents an action. For example, + is an operator that represent addition. An operator works on two or more operands and produce an output.
Types:
1. Arithmetic Operators:
+ is for addition.
- is for subtraction.
* is for multiplication
/ is for division.
% is for modulo.
2. Assignment Operators:
=, +=, -=, *=, /=, %=
3. Auto-increment and auto-decrement operators:
++ and - -
4. Logical operators:
&&, ||, !
5. Relational operators:
==, !=, >, <, >=, <=
6. Bitwise operators:
&, |, ^, ~, <<, >>
Conditional Statements:
IF: The if statement evaluates the test expression inside the parenthesis.
- If the test expression is evaluated to true, statements inside the body of if is executed.
- If the test expression is evaluated to false, statements inside the body of if is skipped from execution.
Syntax: if(TestExpression)
{
Statements;
}
IF...ELSE: If test expression is evaluated to true,
- statements inside the body of if statement is executed.
- statements inside the body of else statement is skipped from execution.
If test expression is evaluated to false,
- statements inside the body of else statement is executed.
- statements inside the body of if statement is skipped from execution
Syntax: if( Expression)
{
Statement;
}
else
{
Statement;
}
Nested IF: It is possible to include if statements inside the body of another if statement. When a series of decision are involved in statement, we use if statement in nested form.
Syntax: if( Expression)
{
if( Expression)
{
Statement;
}
}
Conditional operator: The conditional operator returns one of two values depending on the result of an expression.
Syntax: (expression 1) ? expression 2 : expression 3
If expression 1 is evaluated to true, then expression 2 is evaluated.
If expression 1 is evaluated to false, then expression 3 is evaluated.
Looping Statements:
For: For loop is a statement which allows code to be repeatedly executed. For loop contains 3 parts initialization, condition and increment or decrements.
Syntax: for( initialization; condition; increment/decrement)
{
loop body;
}
While: In while loop first check the condition if condition is true then control goes inside the loop body other wise goes outside the body.
Syntax: While( condition)
{
loop body;
increment/decrement;
}
Do...While: A do..while loop is similar to a while loop, except that a do..while loop is execute at least one time. A do..while loop is a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given condition at the end of the block.
Syntax: do
{
Statements;
increment/decrement;
}while();
Nested loop: A loop can be nested inside of another loop. C++ allows at least 256 levels of nesting.
Syntax: The syntax for a nested for loop.
for( init; condition; increment){
for( init; condition; increment){
statements;
}
statements;
}
The syntax for a nested while loop.
while( condition) {
while( condition) {
statements;
}
statements;
}
The syntax for a nested do..while loop.
do {
statements;
do {
statements;
}while( condition);
}while( condition);
Loop control statements:
Break: When a break statement is encountered inside a loop, the loop is immediately terminated and the program control resumes at the next statement following the loop.
Syntax: break;
Continue: The continue statement skips statements after it inside the loop.
Syntax: continue;
Go to: Go to statement is used for altering the normal sequence of program execution by transferring control to some other part of the program. The go to statement can be used to jump from anywhere to anywhere within a function.
Syntax: goto label1;
..........................
..........................
label1:
statements;
...........................
Functions: A function is a group of statements that together perform a task. A function declaration tells the compiler about a function's name, return type, and parameters. A function definition provides the actual body of the function.
There are two types of functions:
1. Library functions: Library functions are the built-in function, programmer can use library function by invoking function directly.
Ex: log(), pow(), sqrt(), abs() etc.
2. User defined functions: C++ allows programmer to define their own function. A user defined function groups code to perform a specific task and that group of code is given a name.
Write a code to display your name with spaces and without.
Code
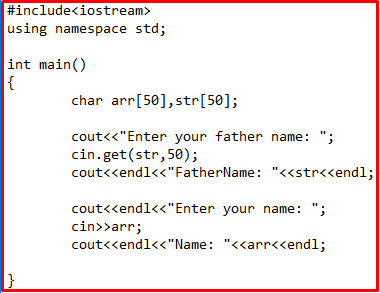
Compile & Run:
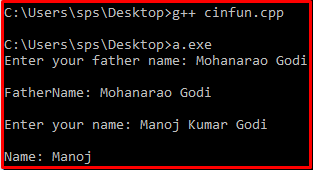
Recursion: The process in which a function calls itself is known as recursion and the corresponding function is called the recursive function.
Ex: factorial function.
Direct recursion: When function calls itself, it is called direct recursion.
Indirect recursion: When function calls another function and that function calls the calling function, then this is called indirect recursion.
Arrays: An array is a series of elements of the same type placed in contiguous memory locations that can be individually referenced by adding an index to a unique identifier.
Why do we need arrays?
We can use normal variables(v1, v2,....vn) when we have small number of objects, but if we want to store large number of instances, it become difficult to manage them with normal variables. The idea of array is to represent many instances in one variable.
Declaration:
1. Declaration by specifying size
Ex: int arr[10];
2. Declaration by initializing elements
Ex: int arr[]={10,20,30}
3. Declaration by specifying size and initialising elements
Ex: int arr[5]={10,20,30,40}
Files:
The iostream standard library provides cin and cout methods for reading from standard input and writing to standard output. To read and write form a file requires another standared C++ library called fstream. Which defines three new data types.
Datatypes:
Ofstream: This data type represents the output file stream and is used to create files and to write information to files.
ifstream: Thsi data type represents the input file stream and is used to read information from files.
fstream: This datatype represents the file stream generally, and has the capabilities of both ofstream and ifstream which means it can create files, write information to files, and read information from files.
Note: To perform file processing in C++, header files <iostream> and <fstream> must be included.
Opening a file: A file must be opened before perform read or write operation on it. Either ofstream or fstream object may be used to open a file for writing. And ifstream object is used to open a file for reading purpose only.
Mode flag:
ios::app : Append mode. All output to that file to be appended to the end.
ios::ate : Open a file for output and move the read/write control to the end of the file.
ios::in : Open a file for reading.
ios::out : Open a file for writing.
ios::trunc : If the file already exists, its contents will be truncated before opening the file.
Closing a file: When a C++ program terminates it automatically flushes all the streams, release all the allocated memory and close all the opened files. But it is always a good practice that should close all the opened files before program termination.
Writing to a file: While doing c++ programming, you write information to a file from your program using the stream insertion operator just as you use that operator to output information to the screen. The only difference is that you use an ofstream or fstream object instead of the cout object.
Reading from a file: You read information from a file into your program using the stream extraction operator just as you use that operator to input information from the keyboard. The only difference is that you use an ifstream or fstream object instead of the cin object.