Variable: In C language, when we want to use some data value in our program, we can store it in a memory space and name the memory space so that it becomes easier to access it. The naming of an address is known as variable. Variable is the name of memory location.
Rules to name a variable:
1. Variable name must not start with a digit. It must begin with either a letter or an underscore.
2. The name of a variable can be composed of letters, digits and the underscore character. 3. Upper and lower case letters are distinct because C is case-sensitive so it is suggested to keep the variable names in lowercase.
4. Blank or spaces are not allowed in variable name.
5. Keywords are not allowed as variable name.
Datatype: A variable in C language must be given a type, which defines what type of data the variable will hold.
1. Char: It store a character data.
2. Int: It store integer data.
3. Float: It store float values.
4. Double: It store double values.
5. Void: It store nothing.
Declaration: Declaration of variables must be done before they are used in the program. Declaration does the following things.
1. It tells the compiler what the variable name is.
2. It specifies what type of data the variable will hold.
3. Until the variable is defined the compiler doesn't have to worry about allocating memory space to the variable.
Initialization: Initializing a variable means to provide it with a value. A variable can be initialized and defined in a single statement like
int a=10;
Declare string variable and assign your name and display your name.
Code:
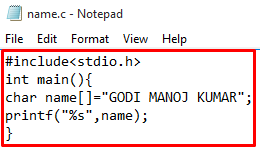
Compile & Run:
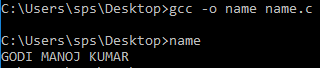
Assign your name to 3 different variables Surname, Firstname, Lastname and display your full name.
Code:
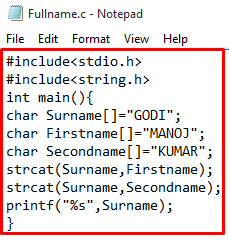
Compile & Run:

Operators: An operator is a symbol which operates on a value or a variable. For example + is an operator to perform addition.
Arithmetic Operators: An arithmetic operator performs mathematical operations such as addition, subtraction and multiplication on numeric values.
ex: +, -, *, /, %.
Relational Operators: A relational operator checks the relationship between two operands. If the relation is true, it returns 1. if the relation is false, it returns 0.
ex: ==, >, <, !=, >=, <=.
Logical Operators: An expression containing logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are commonly used in decision making in C programming.
ex: &&, ||, !.
Bitwise Operators: During computation, mathematical operations like: addition, subtraction, multiplication and division are converted to bit level which makes processing faster. Bitwise operators are used in C programming to perform bit level operations.
ex: &, |, ^, ~, <<, >>.
Assignment Operators: An assignment operator is used for assigning a value to a variable. The most common assignment operator is =.
ex: =, +=, -=, *=, /=, %=.
Conditional Operators:
Syntax: ConditionalExpression ? expression1 : expression2
if ConditionalExpression is true, expression1 is evaluated.
if ConditionalExpression is false, expression2 is evaluated.
Display sum of two numbers:
Code:
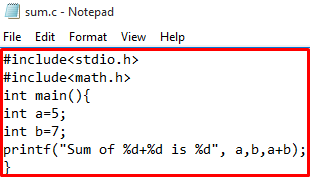
Compile & Run:
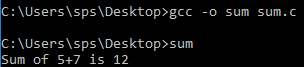
Display highest number in the above two numbers:
Code:
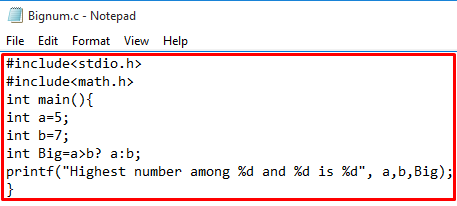
Compile & Run:
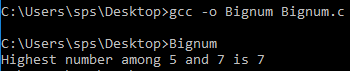
Printf(): In C programming language, printf() function is used to print the character, string, float, integer, octal and hexadecimal values onto the output screen.
Printf() function with %d format specifier to display the value of an integer value.
%s is for string value.
%c is for character value.
%f is for float value.
%lf is for double value.
%x is for hexadecimal value.
Scanf(): In C programming language, scanf() function is used to read character, string, numeric data from keyboard.
Syntax: scanf(%c, &ch);
Ask the user to enter two numbers and display the sum of the two numbers.
Code:
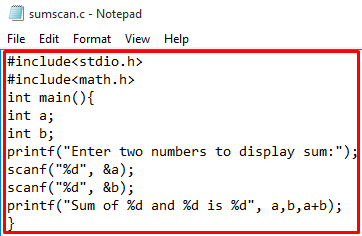
Compile & Run:
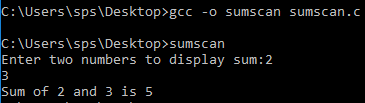
Find the highest of the given two numbers and display it.
Code:
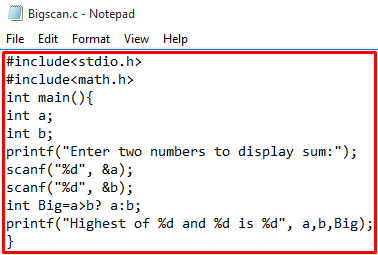
Compile & Run:
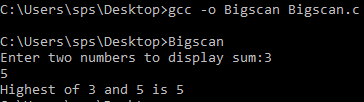
Rules to name a variable:
1. Variable name must not start with a digit. It must begin with either a letter or an underscore.
2. The name of a variable can be composed of letters, digits and the underscore character. 3. Upper and lower case letters are distinct because C is case-sensitive so it is suggested to keep the variable names in lowercase.
4. Blank or spaces are not allowed in variable name.
5. Keywords are not allowed as variable name.
Datatype: A variable in C language must be given a type, which defines what type of data the variable will hold.
1. Char: It store a character data.
2. Int: It store integer data.
3. Float: It store float values.
4. Double: It store double values.
5. Void: It store nothing.
Declaration: Declaration of variables must be done before they are used in the program. Declaration does the following things.
1. It tells the compiler what the variable name is.
2. It specifies what type of data the variable will hold.
3. Until the variable is defined the compiler doesn't have to worry about allocating memory space to the variable.
Initialization: Initializing a variable means to provide it with a value. A variable can be initialized and defined in a single statement like
int a=10;
Declare string variable and assign your name and display your name.
Code:
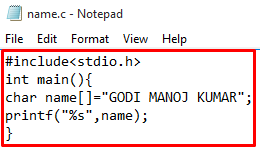
Compile & Run:
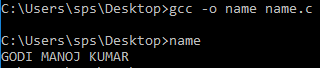
Assign your name to 3 different variables Surname, Firstname, Lastname and display your full name.
Code:
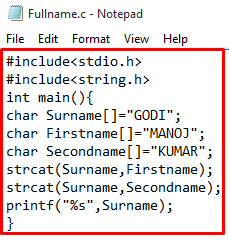
Compile & Run:

Operators: An operator is a symbol which operates on a value or a variable. For example + is an operator to perform addition.
Arithmetic Operators: An arithmetic operator performs mathematical operations such as addition, subtraction and multiplication on numeric values.
ex: +, -, *, /, %.
Relational Operators: A relational operator checks the relationship between two operands. If the relation is true, it returns 1. if the relation is false, it returns 0.
ex: ==, >, <, !=, >=, <=.
Logical Operators: An expression containing logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are commonly used in decision making in C programming.
ex: &&, ||, !.
Bitwise Operators: During computation, mathematical operations like: addition, subtraction, multiplication and division are converted to bit level which makes processing faster. Bitwise operators are used in C programming to perform bit level operations.
ex: &, |, ^, ~, <<, >>.
Assignment Operators: An assignment operator is used for assigning a value to a variable. The most common assignment operator is =.
ex: =, +=, -=, *=, /=, %=.
Conditional Operators:
Syntax: ConditionalExpression ? expression1 : expression2
if ConditionalExpression is true, expression1 is evaluated.
if ConditionalExpression is false, expression2 is evaluated.
Display sum of two numbers:
Code:
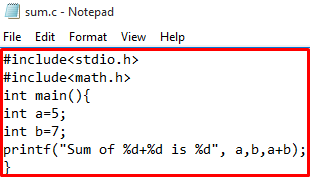
Compile & Run:
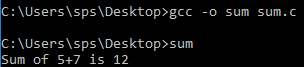
Display highest number in the above two numbers:
Code:
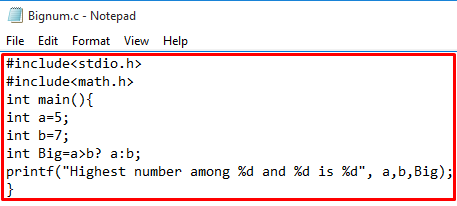
Compile & Run:
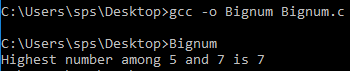
Printf(): In C programming language, printf() function is used to print the character, string, float, integer, octal and hexadecimal values onto the output screen.
Printf() function with %d format specifier to display the value of an integer value.
%s is for string value.
%c is for character value.
%f is for float value.
%lf is for double value.
%x is for hexadecimal value.
Scanf(): In C programming language, scanf() function is used to read character, string, numeric data from keyboard.
Syntax: scanf(%c, &ch);
Ask the user to enter two numbers and display the sum of the two numbers.
Code:
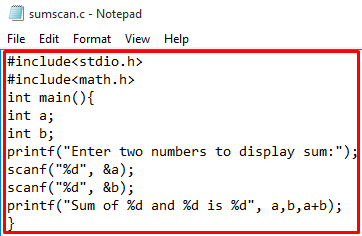
Compile & Run:
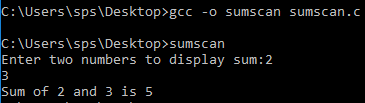
Find the highest of the given two numbers and display it.
Code:
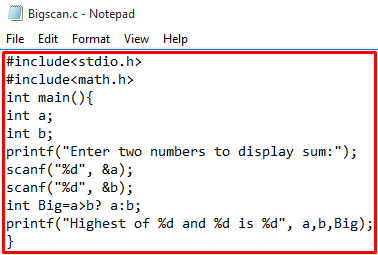
Compile & Run:
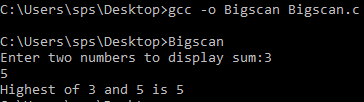