An array is a collection of data items, all of the same type, accessed using a common name. A one dimensional array is like a list. A two dimensional array is like a table.
Declaring: Array variables are declared identically to variables of their data type, except that the variable name is followed by one pair of square brackets for each dimension of the array.
Ex: int arr[10];
Initialising: Arrays may be initialised when they are declared. Place the initialisation data in curly {} braces following the equal sign. An array may be partially initialised by providing fewer data items than the size of the array. The remaining array elements will be automatically initialised to zero.
Ex: int arr[10]={ 1, 2, 3, 4, 5, 6, 7}
Note: Array indices start at zero and go to one less than the size of the array.
Multidimensional array: Multi dimensional arrays are declared by providing more than one set of square brackets after the variable name in the declaration statement. For two dimensional arrays, the first dimension is commonly considered to be the number of rows, and the second dimension the number of columns.
Ex: int arr[2][3]={{1, 2, 3}, {4, 5, 6}}
Accessing: An element in a two dimensional array is accessed by using the subscripts, i.e, row index and column index of the array.
Ex: int value=arr[1][2];
Write a array to display our team names.
Code:
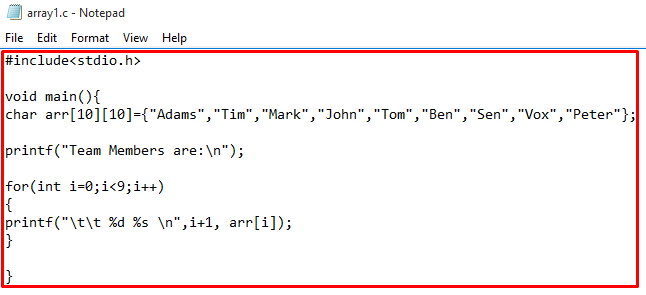
Compile & Run:
Multidimensional array to display name and number.
Code:
Compile & Run:
Write a program to display highest repeated letter in a string.
Code:
Compile & Run:
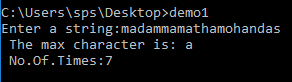
File:
File is used to store the data. There are two types of files. They are text files and binary files.
1. Text files: It can be created easily using notepad or any simple text editors. When you open those files, you'll see all the contents within the file as plain text. You can easily edit or delete the contents. Text files provide least security and takes bigger storage space.
2. Binary files: Binary files are mostly the .bin files in your computer. Instead of storing data in plain text, they store it in the binary form. They are not readable easily and provides a better security.
File Modes:
1. r: Open for reading.
2. rb: Open for reading in binary mode.
3. w: Open for writing.
4. wb: Open for writing in binary mode.
5. a: Open for append. i.e., Data is added to end of file.
6. ab: Open for append in binary mode.
7. r+: Open for both reading and writing.
8. rb+: Open for both reading and writing in binary mode.
9. w+: Open for both reading and writing.
10. wb+: Open for both reading and writing in binary mode.
11. a+: Open for both reading and appending.
12. ab+: Open for both reading and appending in binary mode.
File Operations: Major file operations are
1. Creating a new file
2. Opening an existing file
3. Closing a file
4. Reading from and writing information to a file.
Creating a new file: Creating a file is performed using function fopen() with file mode is w.
Ex: fopen("newfile.txt","w");
Opening an existing file: Opening a file is performed using the library function fopen().
Ex: fptr= fopen("filename","mode");
Closing a file: The file should be closed after reading/writing. Closing a file is performed using library function fclose().
Ex: fclose(fptr);
Reading from a file:
Text File:
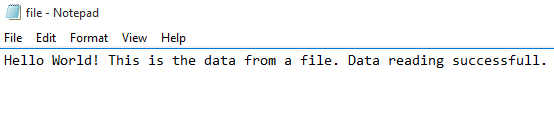
Code:
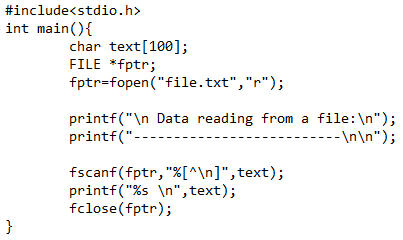
Compile & Run:
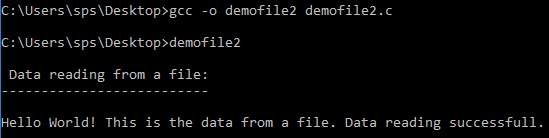
Writing data into a file:
Code:
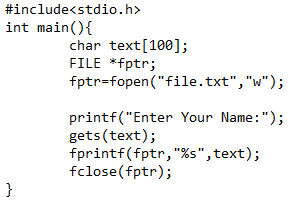
Compile & Run:

Text File:
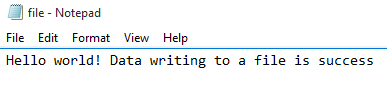
Write your name to a text file and read from text file and display it.
Code:
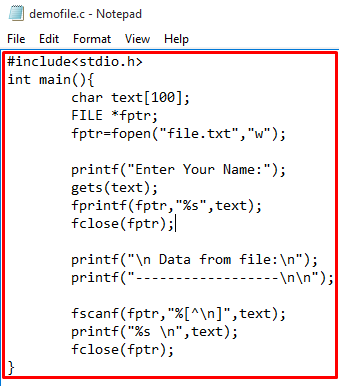
Compile & Run:
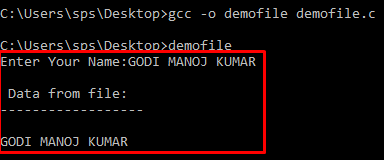
Ex: int arr[2][3]={{1, 2, 3}, {4, 5, 6}}
Accessing: An element in a two dimensional array is accessed by using the subscripts, i.e, row index and column index of the array.
Ex: int value=arr[1][2];
Write a array to display our team names.
Code:
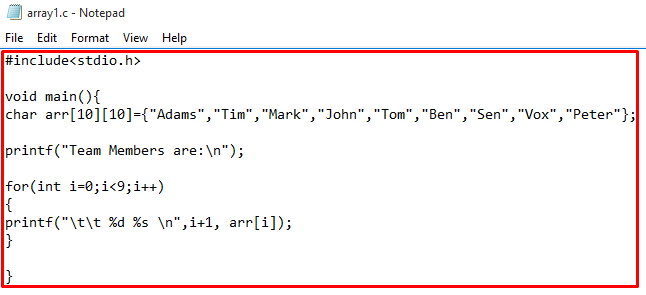
Compile & Run:
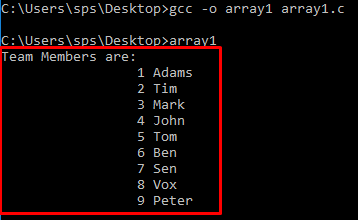
Multidimensional array to display name and number.
Code:
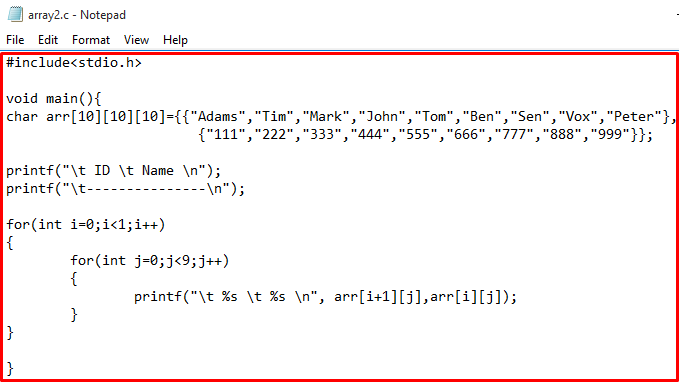
Compile & Run:
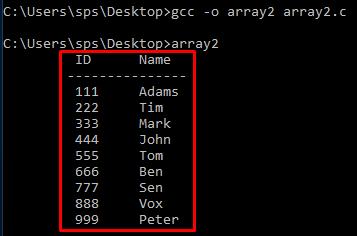
Write a program to display highest repeated letter in a string.
Code:
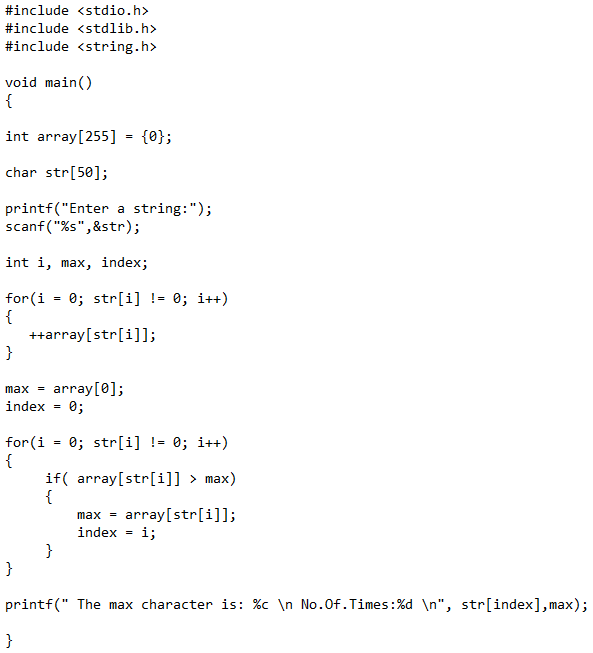
Compile & Run:
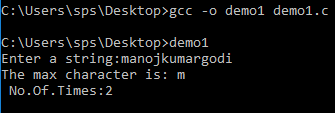
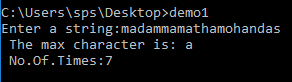
File:
File is used to store the data. There are two types of files. They are text files and binary files.
1. Text files: It can be created easily using notepad or any simple text editors. When you open those files, you'll see all the contents within the file as plain text. You can easily edit or delete the contents. Text files provide least security and takes bigger storage space.
2. Binary files: Binary files are mostly the .bin files in your computer. Instead of storing data in plain text, they store it in the binary form. They are not readable easily and provides a better security.
File Modes:
1. r: Open for reading.
2. rb: Open for reading in binary mode.
3. w: Open for writing.
4. wb: Open for writing in binary mode.
5. a: Open for append. i.e., Data is added to end of file.
6. ab: Open for append in binary mode.
7. r+: Open for both reading and writing.
8. rb+: Open for both reading and writing in binary mode.
9. w+: Open for both reading and writing.
10. wb+: Open for both reading and writing in binary mode.
11. a+: Open for both reading and appending.
12. ab+: Open for both reading and appending in binary mode.
File Operations: Major file operations are
1. Creating a new file
2. Opening an existing file
3. Closing a file
4. Reading from and writing information to a file.
Creating a new file: Creating a file is performed using function fopen() with file mode is w.
Ex: fopen("newfile.txt","w");
Opening an existing file: Opening a file is performed using the library function fopen().
Ex: fptr= fopen("filename","mode");
Closing a file: The file should be closed after reading/writing. Closing a file is performed using library function fclose().
Ex: fclose(fptr);
Reading from a file:
Text File:
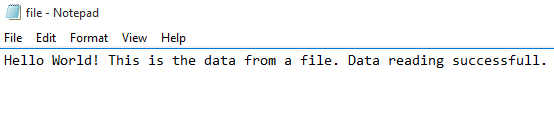
Code:
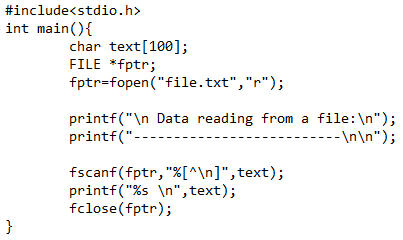
Compile & Run:
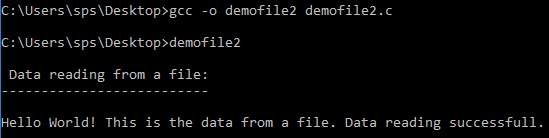
Writing data into a file:
Code:
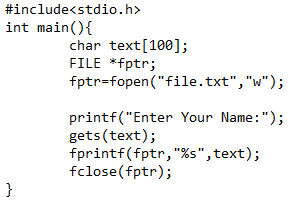
Compile & Run:

Text File:
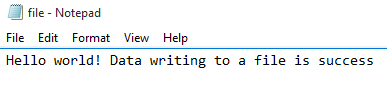
Write your name to a text file and read from text file and display it.
Code:
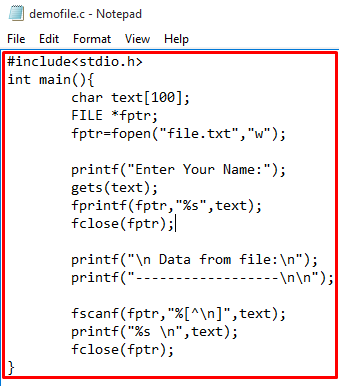
Compile & Run:
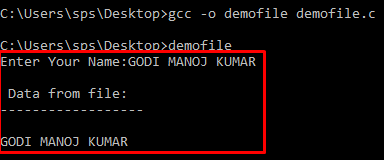